PDP-11/40 Test Programmes
Here is a list of small test programs to run on the PDP-11/40 to verify its basic functions.
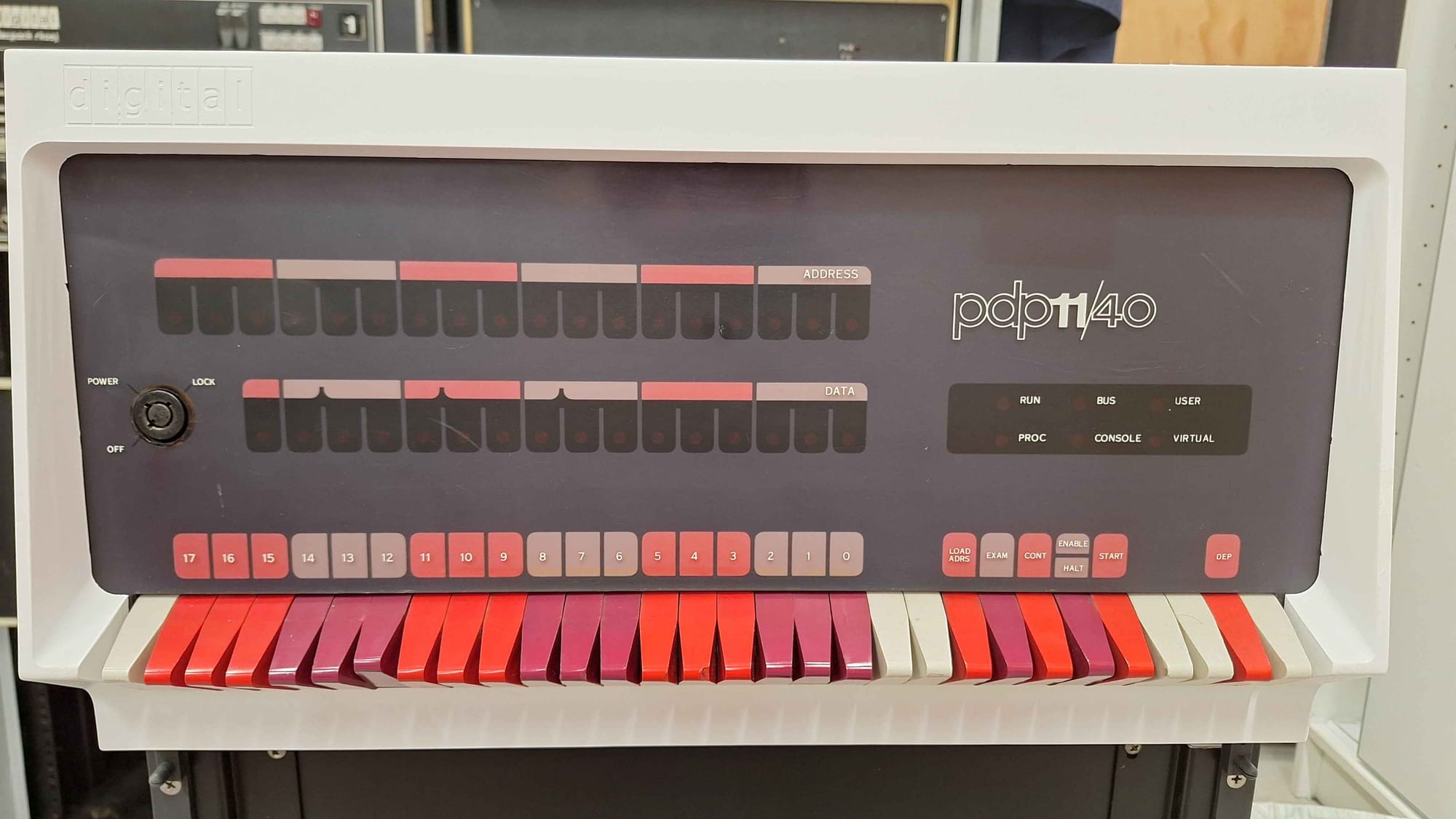
Here is a list of small test programs to run on the PDP-11/40 to verify its basic functions. These programs are not exhaustive.
These programmes have been built with macro11.exe using the listing flag.
To aid in testing the PDP-11 I found a PDP-11 emulator project written in JS. I have forked that project and have hacked in some useful buttons, you can find it here: https://pdp.tephra.me/
Endless Loop
This is a loop that will clear R0 over and over forever. This program is best ran by single-stepping, you should see the addresses 001000, 001002, 001004 repeat over and over.
Address Data Code Switch commands
HALT, 001000, LOAD ADDRESS
001000 005000 clr r0 012700, DEPOSIT
001002 005200 000707, DEPOSIT
001004 000775 br .-4 000775, DEPOSIT
001000, LOAD ADDRESS, ENABLE, START
Single-stepping an endless loop
Increment Loop
This is a simple INC in a loop.
Address Data Code Switch commands
HALT, 001000, LOAD ADDRESS
001000 005000 clr r0 012700, DEPOSIT
001002 005200 000707, DEPOSIT
001004 000005 inc r0 001004, DEPOSIT
001004 000775 br .-4 000775, DEPOSIT
001000, LOAD ADDRESS, ENABLE, START
Increment Loop
Light Chaser
This is a simple program that will display a simple bit pattern on the screen and shift it left in a loop.
Address Data Code Switch commands
HALT, 001000, LOAD ADDRESS
001000 012700 mov #1,r0 012700, DEPOSIT
001002 000707 000707, DEPOSIT
001004 006100 rol r0 006100, DEPOSIT
001006 000005 reset 000005, DEPOSIT
001010 000775 br .-4 000775, DEPOSIT
001000, LOAD ADDRESS, ENABLE, START
Light Chaser
Debugging
In my system, there was a fault with the rotate left (ROL) instruction. When single-stepping the program it would break out of the console state machine and start running but out of control. The rotate left instruction mainly depends on two boards, the M7231 - Data Paths and M7233 - IR Decode.
The M7231 - Data Paths board contains the ALU and is needed for the shift. The M7233 - IR Decode is used to decode instructions and provides control of the Arithmetic Logic Unit. In my system, the M7233 - IR Decode had failed and a replacement fixed the issue.
Copy Cat
1 .ASECT
2 001000 .=1000
3 001000 013700 177570 INIT: MOV @#777570,R0 ;; comment
4 001004 000005 RESET
4 001006 000774 BR .-6
001000, LOAD ADDRESS, ENABLE, START
AAAAAAAAAAAAAAAAAAAA
This basic program prints the letter "A" to the console in a loop.
This program has been made as simple as possible. It needs a DL11 installed at 177560.
1 .ASECT
2 002000 .=2000
3
4 wait:
5 002000 105737 177564 tstb @#177564
6 002004 100375 bpl wait
7 002006 112737 000101 177566 movb #101, @#177566
8 002014 000771 br wait
8
Addressing Modes
Here is a collection of very small programs to test addressing modes. These samples primarily use the INC instruction because it doesn't require any additional setup, unlike the ADD instruction.
1 .ASECT
2 ; Initialise memory to 0
3
4
5 ; Autoincrement - 2000-2010 will INC by one
6 001000 .=1000
7 001000 012700 002000 MOV #2000, R0 ; Load 2000 in R0
8 001004 005220 INC (R0)+ ; INC 2000
9 001006 005220 INC (R0)+ ; INC 2002
10 001010 005220 INC (R0)+ ; INC 2004
11 001012 005220 INC (R0)+ ; INC 2006
12 001014 005220 INC (R0)+ ; INC 2010
13
14
15 ; Autodecrement - 2010-2000 will INC by one
16 001000 .=1000
17 001000 012700 002010 MOV #2010, R0 ; Load 2000 in R0
18 001004 005240 INC -(R0) ; INC 2010
19 001006 005240 INC -(R0) ; INC 2006
20 001010 005240 INC -(R0) ; INC 2004
21 001012 005240 INC -(R0) ; INC 2002
22 001014 005240 INC -(R0) ; INC 2000
23
24
25 ; Index - 2002 will be INC by one
26 001000 .=1000
27 001000 012700 002000 MOV #2000, R0 ; Load 2000 in R0
28 001004 005260 000002 INC 2(R0) ; INC value pointed to by (R0) + 2 (2002)
29
30
31 ; Register Deferred - INC 2000 by one
32 001000 .=1000
33 001000 012700 002000 MOV #2000, R0 ; Load 2000 in R0
34 001004 005210 INC @R0 ; INC 2000
35
36
37 ; Autoincrement Deferred - Set 2000 to 2000 and INC, Set 2002 to 2002 and INC
38 001000 .=1000
39 001000 012700 002000 MOV #2000, R0 ; Load 2000 in R0
40 001004 012737 002000 002000 MOV #2000, @#2000
41 001012 012737 002002 002002 MOV #2002, @#2002
42 001020 005230 INC @(R0)+ ; INC 2000
43 001022 005230 INC @(R0)+ ; INC 2002
44 001024 000777 BR . ; Endless loop
45
46
47 ; Autodecrement Deferred - Set 2000 to 2000 and INC, Set 2002 to 2002 and INC
48 001000 .=1000
49 001000 012700 002004 MOV #2004, R0 ; Load 2004 in R0
50 001004 012737 002000 002000 MOV #2000, @#2000
51 001012 012737 002002 002002 MOV #2002, @#2002
52 001020 005250 INC @-(R0) ; INC 2000
53 001022 005250 INC @-(R0) ; INC 2002
54 001024 000777 BR . ; Endless loop
55
56
57 ; Index Deferred - 1014 will be INC by one
58 001000 .=1000
59 001000 012700 001010 MOV #1010, R0
60 001004 005270 000002 INC @2(R0) ; (R0 + 2) is the pointer to the value to increment
61 001010 000777 BR .
62 001012 001014 .+2 ; Pointer to value to increment
63 001014 000000 0 ; Will be incremented
64
65
66 ; Relative
67 001000 .=1000
68 001000 005267 000002' INC 2 ; PC + 2 bytes (PC = . + 2)
69 001004 000777 BR . ; 2 bytes
70 001006 000000 0 ; Will be incremented
71
72 ; Relative Deferred - 001010 is incremented
73 001000 .=1000
74 001000 005277 000002' INC @2 ; (PC + 2) is the pointer to the value to increment
75 001004 000777 BR .
76 001006 001010 .+2 ; Pointer to value to increment
77 001010 000000 0 ; Will be incremented
78
78